How can you learn Flutter
A Comprehensive Guide to Learning Flutter: How to Get Started and Become a Professional App Developer
Introduction:
Flutter is an open-source framework developed by Google for building mobile, web, and desktop applications using a single codebase. It allows you to easily create cross-platform applications, making it possible to develop apps for Android, iOS, web, and desktop using the same code. Flutter is based on the Dart language, which offers high performance thanks to its ability to be compiled into native code.
If you're interested in learning Flutter and building flexible, beautiful apps, here's a comprehensive guide to help you get started.
Why Learn Flutter?
- Cross-Platform Development
A key feature of Flutter is its ability to build apps that work across multiple platforms with a single codebase. You only need to write the code once, and you can run it on Android, iOS, web, and desktop systems. This way, you save time and effort compared to writing separate code for different platforms.
- High Performance
Flutter uses the Dart language, which is compiled into native code, making apps perform incredibly fast compared to many other frameworks. The code is compiled directly, which means performance is not affected when interacting with complex user interfaces.
- Flexible and Beautiful Design
Flutter provides a wide range of tools that allow you to build rich, sophisticated user interfaces. Using Flutter, you can create highly interactive interfaces with minimal effort, with support for rich graphics and smooth interactions.
- Strong Community and Comprehensive Resources
Flutter is backed by Google and has a large community of developers, making it easy to find answers to any questions you may have. There are also numerous tutorials, articles, and open-source projects that you can join or contribute to.
Steps to Learn Flutter
- Learn the Basics of Dart
Before you start using Flutter, it's essential to learn the basics of Dart, as it is the primary programming language used in Flutter. Dart is a powerful, easy-to-learn language that is fully compatible with the requirements of app development in Flutter.
Suggested Resources for Learning Dart:
- Official Dart tutorials
- Video courses on YouTube
- Codecademy Dart course
Example Dart Code:
In this simple example, we created a variable greeting
containing the text "Welcome to Flutter!" and printed it using print()
.
- Set Up the Development Environment
Before you start using Flutter, you need to set up the development environment. Here's how to install the Flutter SDK and Dart.
Installation Steps:
- Download the Flutter SDK from flutter.dev.
- Install a code editor like Visual Studio Code or Android Studio.
- Install the Flutter and Dart plugins in your editor.
Suggested Resources:
- Flutter Installation Guide
- Getting Started with Flutter: Building Your First App
Now that you have set up the development environment, you can begin building a simple app with Flutter.
Example of a Simple Flutter App:
This code creates a simple app with a title bar and a text in the center of the screen. MaterialApp
is the root widget used to set up the basic design of the app.
- State Management in Flutter
One important task when working with Flutter is learning state management. Flutter provides several ways to manage app state, such as Provider
, setState
, Riverpod
, and Bloc
.
Example Using setState
to Manage State:
In this example, we created an app that displays a counter that can be increased using setState
. This is the basic method for managing state in Flutter.
- Build Practical Projects
One of the best ways to learn Flutter is by building practical projects. You can start by building small apps to apply what you've learned.
Examples of Flutter Projects:
- To-Do List app
- News app that fetches articles using an API
- Simple chat app using Firebase
Suggested Resources:
- Flutter Challenges on GitHub
- Flutter Projects on Medium
- Optimize Performance in Flutter
Optimizing app performance is important, especially when you start building complex apps. Flutter provides tools for measuring and analyzing performance.
Example for Performance Optimization:
Use ListView.builder
instead of a regular ListView
to load items dynamically only when they are visible.
Example Using ListView.builder
:
Here, we use ListView.builder
to dynamically display a list of items, which improves performance when the list contains many elements.
- Interacting with APIs in Flutter
One of Flutter's great features is its ability to interact with APIs to display data from the internet or send data to servers. You can use the http
package to make HTTP requests.
Example of Interacting with an API in Flutter:
Conclusion
Learning Flutter is a great investment for your future as an app developer, thanks to its ability to build cross-platform apps using a single codebase. With the continuous development of Flutter and its support from Google, it has become the ideal choice for modern, diverse app development. By committing to practice and continuous learning, you can sharpen your Flutter skills and create amazing applications.
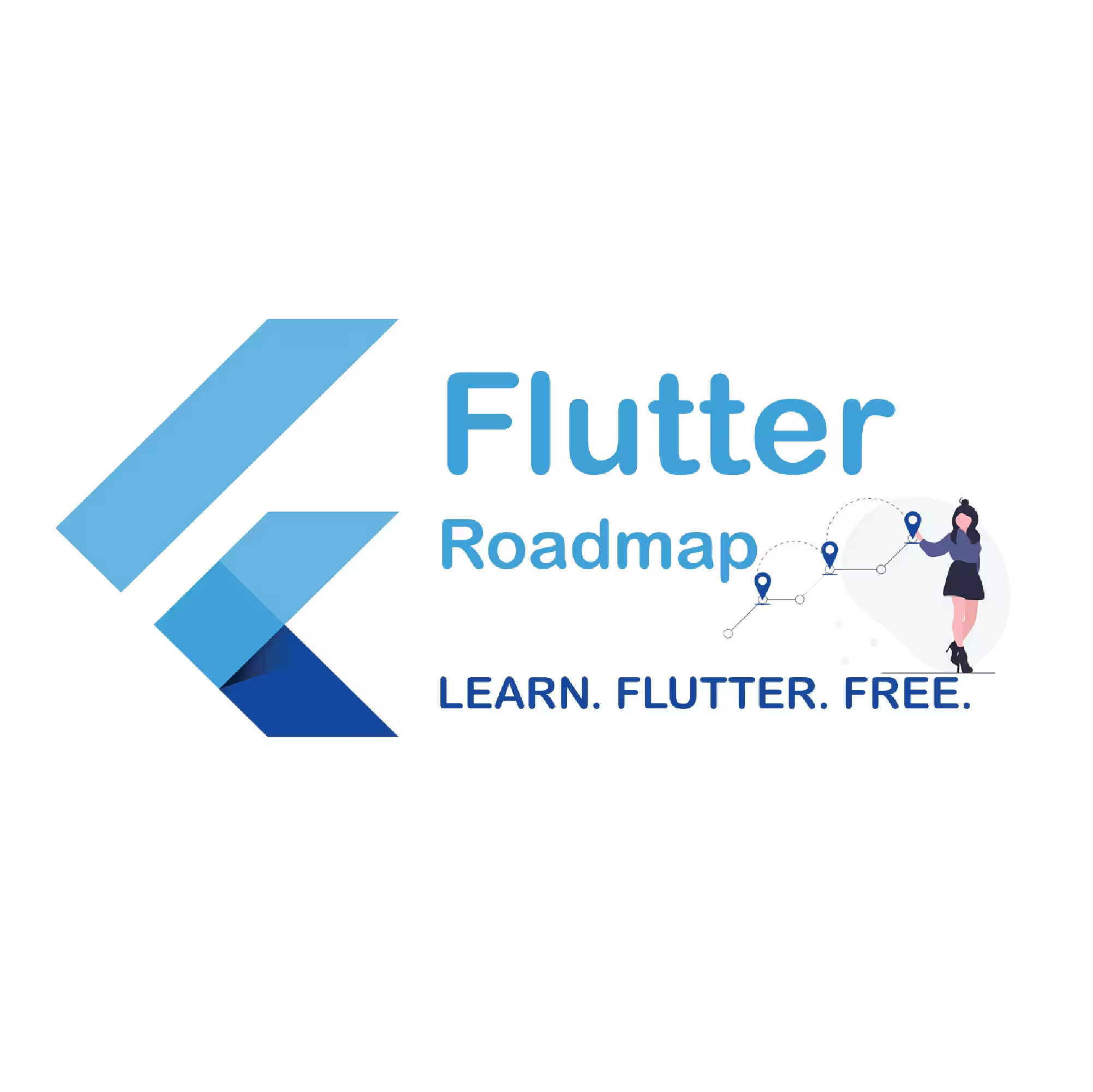